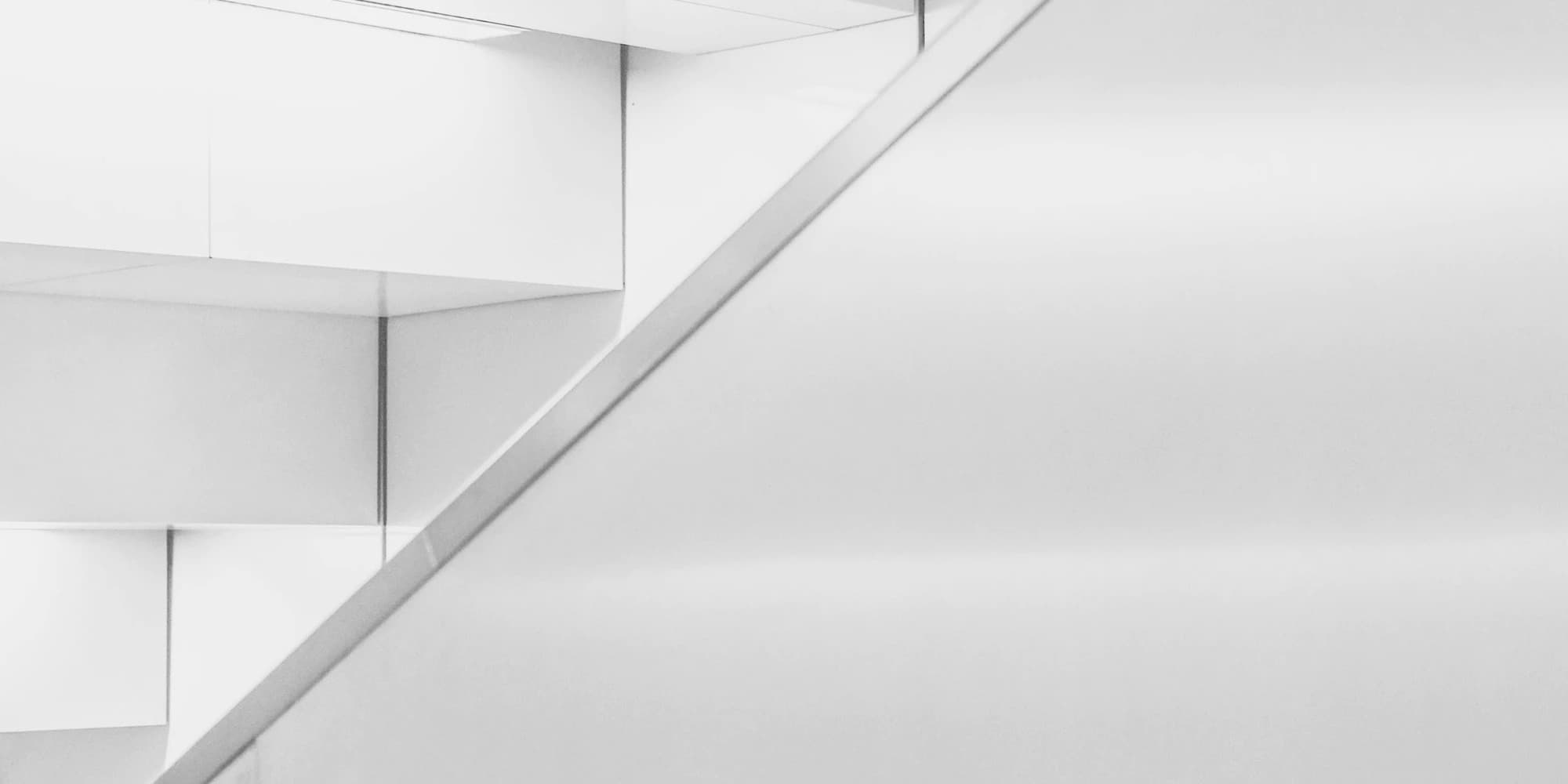
AI-Assisted Coding with Cursor
How to read this guide: This document offers two reading paths - bold text provides a concise overview of core concepts, while the regular text offers additional details and examples. You can either follow the bold path for a quick understanding or read everything for a comprehensive view. You can also select all the text, right-click, copy it as markdown, and then feed it to a model to understand specific parts of the text.
Using Cursor to Assist with Your Code {#using-cursor-to-assist-with-your-code}
Cursor is an AI-assisted code editor with chat-based assistance built in. This guide focuses on practical techniques for effective usage.
Core Commands for Daily Use {#core-commands-for-daily-use}
Getting started with Cursor is straightforward - use these essential commands:
- Open chat panel:
Cmd+L
(Mac) orCtrl+L
(Windows/Linux) - Basic operation: Type natural language questions or commands
- Navigate UI: Use standard editor shortcuts plus AI-specific commands
Using @ Commands Effectively {#using-@-commands-effectively}
The key to productive Cursor usage is providing proper context with @ commands:
@Files
- Add specific files as context
// When you need help with a specific implementation
Fix the authentication bug in @Files auth/authService.js
@Folders
- Include entire directories as context
// When you need broader understanding
How do the components in @Folders src/components/dashboard interact?
@Code
- Reference code blocks or functions
// When referencing specific functions or patterns
Create a similar function to @Code function validateUserInput
@Web
- Reference web documentation
// When you need external standards information
What's the best way to implement forms according to @Web react.dev/reference/hooks?
@Linter
- Reference lint warnings and errors
Pro tip: Combine multiple @ commands in a single prompt to provide comprehensive context.
Introduction: Engineering at a Strategic Level with AI {#introduction:-engineering-at-a-strategic-level-with-ai}
AI coding assistants are changing how software engineers work. By delegating certain implementation tasks to AI, you can shift some of your focus toward higher-level concerns—addressing bigger scopes and shipping more quality products.
This shift is gradually changing aspects of the engineer's role from hands-on coding of every detail to a mix of direct implementation and oversight:
- Broader context - Handle more aspects of a project with AI assistance on implementation details
- Design focus - Spend more time on architecture and design decisions, with less time on boilerplate
- Enhanced quality - Explore more edge cases and testing scenarios than time would typically allow
- Improved productivity - Complete certain tasks more efficiently, particularly routine implementations
This comprehensive guide explores practical approaches to integrating AI into your development workflow:
- Understanding how AI models process code and context to improve your interactions
- Developing effective communication techniques for iterative refinement with AI
- Building structured domain-specific knowledge to enhance AI's understanding of your codebase
- Organizing and federating rules for maintaining knowledge at scale
- Creating workflow patterns that maximize AI strengths while maintaining human oversight
- Implementing advanced techniques for complex projects across multiple technologies
- Using persona-based testing to uncover edge cases before implementation
- Troubleshooting common challenges in AI coding sessions
The engineers making the most of AI tools aren't just using them to automate simple tasks—they're integrating them into all their processes, redirecting their attention to areas where human expertise adds the most value.
While AI tools are improving, they remain assistive rather than autonomous, requiring guidance, producing errors, and struggling with complex architectural decisions. Success depends on realistically assessing what to delegate versus what needs human oversight, while maintaining core engineering skills of problem-solving, attention to detail, and quality.
The Three-Step Engineering Process {#the-three-step-engineering-process}
Great software engineering has always followed a pattern, whether you're working alone or with AI:
1. Find the Problem
2. Break it Down
3. Solve It
Let's explore how AI transforms each step.
Finding the Problem {#finding-the-problem}
AI can significantly enhance your ability to understand and define problems:
- Explore unfamiliar domains - Have AI explain complex concepts, industry standards, or patterns using
@Web
to reference authoritative sources - Navigate large codebases - Ask questions about architecture, dependencies, and code flow with
@Files
and@Folders
for broader context - Identify blind spots - Explore potential edge cases or alternative perspectives
- Gather context - Combine documentation, existing code (
@Code
), and external references
For example, when exploring a payment processing system, you might ask: "Explain how credit card authorization flows work and what the key security considerations are."
Breaking it Down {#breaking-it-down}
Once you understand the problem, AI excels at decomposition:
- Task identification - Breaking large features into specific implementation tasks
- Dependency mapping - Understanding relationships between components through code exploration
- Estimating complexity - Helping gauge the difficulty of different approaches
- Alternative solutions - Exploring various implementation strategies by referencing existing patterns with
@Code
When starting a new feature, try prompts like: "Let's break down implementing an expense approval workflow. What components and data structures will we need?"
Solving It {#solving-it}
This is where AI collaboration really shines:
- Planning - Outlining implementation steps before writing code
- Implementation - Generating code based on requirements while referencing similar patterns with
@Code
- Testing strategies - Creating comprehensive test plans and addressing
@Lint Errors
proactively - Documentation - Automating documentation of your solutions
The key difference with AI-assisted implementation is the importance of upfront planning. While traditional coding often involves figuring out the next step as you implement, AI works better with a clear roadmap from the beginning.
How AI Models Understand Code and Context {#how-ai-models-understand-code-and-context}
To collaborate effectively with AI, it helps to understand how these systems process information:
Mental Models and Knowledge Representation {#mental-models-and-knowledge-representation}
AI language models organize knowledge in semantic clusters—related concepts and patterns grouped together. When you prompt an AI, you're activating specific knowledge areas.
This is why precise, domain-specific language helps. When you use terms like "GraphQL resolver" or "dependency injection pattern," you're directing the AI to the right knowledge clusters.
Two Key AI Capabilities to Leverage {#two-key-ai-capabilities-to-leverage}
1. Chain-of-Thought Reasoning {#1.-chain-of-thought-reasoning}
AI works better when allowed to reason step by step through complex problems. Compare these approaches:
Basic prompt:
Implement a pagination system for our API.
Chain-of-thought prompt:
Let's implement pagination for our API. We'll need to:
1. Decide between cursor-based or offset pagination
2. Define how page size is controlled
3. Implement the database query logic
4. Create the response format with links to next/previous pages
The second approach helps the AI organize its thinking and produce more coherent solutions. You can enhance this by using @Files
to reference existing implementation patterns in your codebase.
2. Needle-in-a-Haystack Filtering {#2.-needle-in-a-haystack-filtering}
Modern AI models excel at extracting relevant information from large amounts of context. This is invaluable when:
- Debugging complex logs by providing context with
@Files
- Understanding large files with focused queries
- Finding specific implementation patterns in a large codebase using
@Recent Changes
to track implementations
For example, you can paste hundreds of lines of logs and ask: "What's causing the authentication failures in these logs?"
An illustrative example of this capability: when the entire script of the Bee Movie was pasted into a chat with two questions embedded within it—"How to center a div?" and "Create a website"—the AI correctly identified and answered both questions despite the overwhelming amount of unrelated text.
This filtering capability is particularly useful when working with verbose logs, large configuration files, or extensive documentation, where finding the relevant information manually would be time-consuming. It allows you to quickly isolate important patterns or issues without manual scanning.
Effective Communication Techniques {#effective-communication-techniques}
The quality of your interaction with AI directly affects the quality of the code you'll produce together. Here are the most effective communication strategies:
Explicit Planning Before Implementation {#explicit-planning-before-implementation}
Instead of jumping straight to implementation, start with a plan:
I need to implement a user profile update feature. Before writing code, let's outline:
1. What endpoints we'll need
2. Data validation requirements
3. Database operations involved
4. Error handling approach
This approach allows you to shape the solution before committing to specific code patterns.
Iterative Refinement {#iterative-refinement}
Once you have an initial plan or implementation, refine it collaboratively:
That implementation looks good, but I have a few adjustments:
1. Let's use JWT for authentication instead of session cookies
2. We should add rate limiting to prevent abuse
3. Let's make sure we validate email format
This iterative approach combines AI's ability to generate code with your judgment about what's appropriate for your specific context.
One of the most significant advantages of AI-assisted development is the dramatically reduced cost of iterations. Traditional development often limits the number of refinement cycles due to time constraints. With AI, you can:
- Run through multiple iterations in the time it would take to complete one manual cycle
- Experiment with alternative approaches to compare different solutions
- Focus on improving quality rather than just meeting minimum requirements
- Progressively enhance implementations with additional edge cases and optimizations
Since revisions are quick and inexpensive, you can pursue higher quality standards through successive refinements that would be impractical in traditional development workflows. Each iteration can focus on different aspects: correctness, performance, security, maintainability, or edge cases, leading to a more comprehensive solution.
Avoiding the One-Shot Trap {#avoiding-the-one-shot-trap}
Many engineers fall into the trap of trying to solve problems in a single prompt. This approach often leads to suboptimal solutions that don't leverage the AI's full capabilities. Instead:
- Embrace incremental progress - Break complex tasks into a series of smaller, focused iterations
- Commit code at meaningful checkpoints - Use version control to save stable iterations, allowing you to roll back if the AI gets confused
- Try different approaches - If one approach isn't working, start a new branch and try an alternative strategy
- Review and refine - Take time to evaluate each iteration before moving forward
Managing Conversations: New vs. Continued {#managing-conversations:-new-vs.-continued}
When should you start a new conversation versus continuing a long-threaded one? This common question involves weighing context against clarity:
-
Continue the same conversation when:
- You're iteratively refining a specific implementation
- Context from earlier in the conversation is crucial
- You're working through closely related aspects of the same feature
- The conversation history helps the AI understand your specific requirements
-
Start a new conversation when:
- The AI has become confused or fixated on an incorrect approach
- You're shifting to a substantially different part of the project
- The conversation has grown too long, affecting response quality or speed
- You want to try a completely fresh approach to the problem
While Cursor provides checkpoint restoration functionality, consider that rolling back checkpoints discards valuable tokens and learning opportunities. Often, the need to use checkpoints indicates that you started implementing too early or haven't provided enough context for the model. Rather than discarding the conversation, try adding more context or creating specific rules that address the confusion.
This iterative approach can transform how teams implement large-scale projects. Features that historically would take multiple quarters and require collaboration from many engineers can be approached more efficiently. The reduced cost of change enables teams to aim for global optimization rather than settling for local maxima.